Photo by Myriam Jessier on Unsplash
在隨手可得的數據時代,資料分析逐漸成為顯學,利用視覺化軟體或是分析處理工具,將蒐集的資料轉變為有用的資訊,來協助使用者進行決策。
Pandas套件則是其中一種常用的資料分析處理工具,它是基於Python程式語言來建立的,非常容易使用且強大,主要應用於單維度(Series)與二維度(DataFrame)的資料處理。
大家可以把Pandas套件想像成是Excel一樣,能夠將儲存的資料進行統計、搜尋或修改等操作,主要有兩種資料結構,分別是Series及DataFrame,本文就先帶大家來認識Series的基本觀念,包含:
- 什麼是Pandas Series
- 建立Pandas Series物件
- 取得Pandas Series資料
- 新增Pandas Series資料
- 修改Pandas Series資料
- 取得Pandas Series資料筆數
- Pandas Series字串運算
- Pandas Series數值運算
一、什麼是Pandas Series
Pandas Series適用於處理單維度或單一欄位的資料,就像是Excel中的某一欄,如下圖:
Pandas Series就像上圖一樣,左側為資料索引,右側為資料內容,不過Pandas Series自動產生的資料索引是從0開始計算,另外也有提供方法(Method),讓使用者可以自行定義。
在開始本文的實作前,首先需利用以下的指令來安裝Pandas套件:
$ pip install pandas
二、建立Pandas Series物件
想要利Pandas Series來儲存單維度的資料,就需要先建立Pandas Series物件,語法如下:
my_series = pandas.Series(資料串列)
範例
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone)
執行結果
上圖執行結果的左側,就是Pandas套件自動產生的資料索引或稱資料編號,右側則是指定的資料內容,如果想要自訂資料索引,可以利用index關鍵字參數(Keyword Argument)來進行設定,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"], index=["p1", "p2", "p3", "p4"]) print(phone)
執行結果
三、取得Pandas Series資料
可以利用資料順序或資料索引值來取得Pandas Series物件中的特定資料,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"], index=["p1", "p2", "p3", "p4"]) print(phone[1]) # 依據資料順序取值(從0開始計算) print(phone["p3"]) # 依據資料索引值取值
執行結果
四、新增Pandas Series資料
想要在既有的Pandas Series物件中新增資料,可以利用append()方法(Method)來達成,這邊要特別注意的是,append()方法(Method)需傳入Pandas Series物件,並且會額外產生一個新的Pandas Series物件,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) data = pd.Series(["Htc", "Oppo"]) # 新增的資料 combined = phone.append(data) print(combined)
執行結果
由於Pandas Series物件都會自動產生資料索引,所以從上面的執行結果可以看到,將兩個Pandas Series物件合併後,會發現資料索引沒有連續的問題,這時候可以在append()方法(Method)中加上「ignore_index = True」設定,來忽略資料索引,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) data = pd.Series(["Htc", "Oppo"]) # 新增的資料 combined = phone.append(data, ignore_index=True) print(combined)
執行結果
要修改Pandas Series物件中的某一筆資料,可以利用資料索引值進行存取後,來修改其中的資料,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) phone[1] = "Oppo" print(phone)
執行結果
如果有自訂的資料索引值,同樣可以依據資料索引值來進行修改,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"], index=["p1", "p2", "p3", "p4"]) phone["p3"] = "Oppo" print(phone)
執行結果
六、取得Pandas Series資料筆數
通常在做資料分析時,都會需要知道載入的資料筆數,這時候可以透過Pandas Series物件的size屬性(Attribute)來取得,如下範例:
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone.size) # 執行結果4
七、Pandas Series字串運算
在文章開頭有提到,Pandas套件就像Excel一樣,能夠對儲存的資料進行處理及操作,最常見的就是字串運算,這邊就擷取幾個Pandas Series物件常用的字串運算方法(Method),包含:
- upper():將字串轉為大寫
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone.str.upper()) # 將字串資料轉換為大寫
執行結果
- lower():將字串轉為小寫
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone.str.lower()) # 將字串資料轉換為小寫
執行結果
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone.str.contains("Sa")) # 搜尋是否包含特定字串
執行結果
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone.str.cat(sep=";")) # 利用自訂分隔符號連接字串
執行結果
import pandas as pd phone = pd.Series(["Apple", "Samsung", "Mi", "Sony"]) print(phone.str.replace("Samsung", "Oppo")) # 將Samsung取代為Oppo
執行結果
另一個Pandas套件最常應用的地方,就是數值的運算,這邊列舉幾個Pandas Series物件常用的數值運算方法(Method),包含:
- max():最大值
import pandas as pd numbers = pd.Series([22, 5, 10, 12, 6, 30]) print(numbers.max()) # 執行結果30
- min():最小值
import pandas as pd numbers = pd.Series([22, 5, 10, 12, 6, 30]) print(numbers.min()) #執行結果5
- sum():總和
import pandas as pd numbers = pd.Series([22, 5, 10, 12, 6, 30]) print(numbers.sum()) #執行結果85
- mean():平均數
import pandas as pd numbers = pd.Series([22, 5, 10, 12, 6, 30]) print(numbers.mean()) # 執行結果14.166666666666666
- nlargetst():最大的n個數值
import pandas as pd numbers = pd.Series([22, 5, 10, 12, 6, 30]) print(numbers.nlargest(2)) # 最大的2個數值
執行結果(左側為資料索引,右側為資料內容)
- nsmallest():最小的n個數值
import pandas as pd numbers = pd.Series([22, 5, 10, 12, 6, 30]) print(numbers.nsmallest(2)) # 最小的2個數值
執行結果(左側為資料索引,右側為資料內容)
九、小結
本文帶大家瞭解Pandas套件的Series資料結構,來處理單一欄位的資料內容,從範例的說明中也可以感受到,利用幾個簡單的方法(Method),就能輕鬆的對資料進行操作,在資料分析上,非常的實用,讀者們可以利用本文的教學,來練習操作手邊的資料吧。
有想要看的教學內容嗎?歡迎利用以下的Google表單讓我知道,將有機會成為教學文章,分享給大家😊
- Python學習資源整理
- [Django教學1]3步驟快速安裝Django網站框架
- [Python爬蟲教學]開發Python網頁爬蟲前需要知道的五個基本觀念
- [Python爬蟲教學]有效利用Python網頁爬蟲幫你自動化下載圖片
- [Python爬蟲教學]7個降低Python網頁爬蟲被偵測封鎖的實用方法
- [Python爬蟲教學]非同步網頁爬蟲使用GRequests套件提升爬取效率的實作技巧
- [Python爬蟲教學]學會使用Selenium及BeautifulSoup套件爬取查詢式網頁
- [Python爬蟲教學]解析如何串接Google Sheet試算表寫入爬取的資料
- [Python爬蟲教學]活用openpyxl套件將爬取的資料寫入Excel檔案
- [Python爬蟲教學]教你如何部署Python網頁爬蟲至Heroku雲端平台
- [Python爬蟲教學]輕鬆學會Python網頁爬蟲與MySQL資料庫的整合方式
- [Python爬蟲教學]整合Python Selenium及BeautifulSoup實現動態網頁爬蟲
- [Python爬蟲教學]Python網頁爬蟲動態翻頁的實作技巧
- [Python爬蟲教學]Python網頁爬蟲結合LINE Notify打造自動化訊息通知服務
- [Python+LINE Bot教學]建構具網頁爬蟲功能的LINE Bot機器人
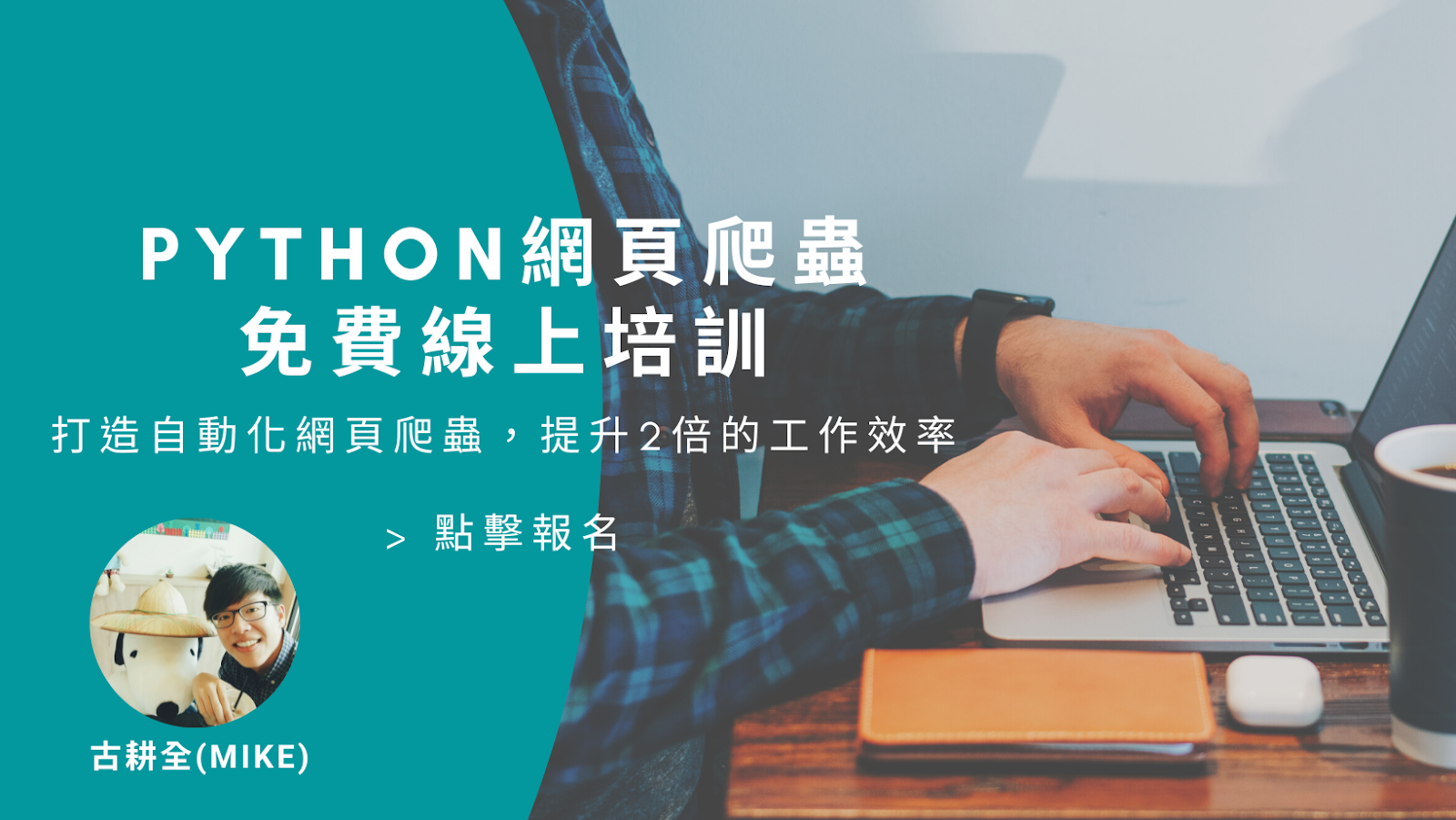
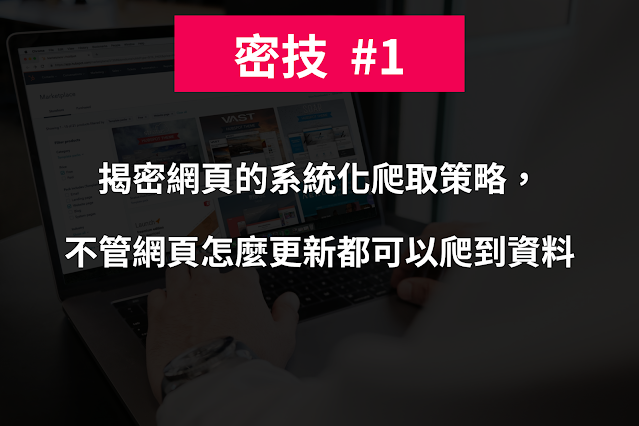
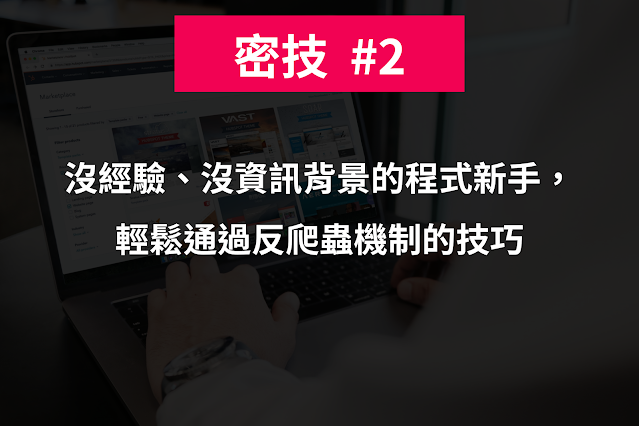
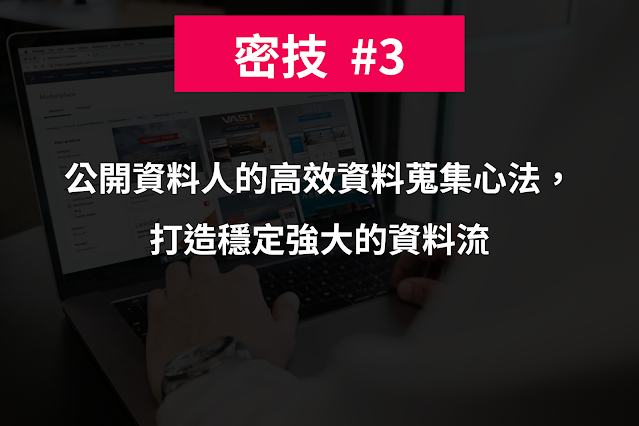
新版本的 Pandas 中,Series 不再支援 append 方法?
回覆刪除combined = pd.concat([phone, data]) # 使用 concat() 替代 append()
刪除